In this post we will create an example of how to get keys and values from a map, using a few approaches.
Suppose we have a map like below:
Map<String, String> map = {
'name': 'Phuc Tran',
'email': 'phuc.tran@nextfunc.com',
'website': 'https://coflutter.com'
};
1. Get all keys from Map
void getKeysFromMap(Map map) {
print('----------');
print('Get keys:');
// Get all keys
map.keys.forEach((key) {
print(key);
});
}
// Output
Get keys:
name
email
website
2. Get all values from Map
void getValuesFromMap(Map map) {
// Get all values
print('----------');
print('Get values:');
map.values.forEach((value) {
print(value);
});
}
// Output
Get values:
Phuc Tran
phuc.tran@nextfunc.com
https://coflutter.com
3. Get all keys and values using forEach method
void getKeysAndValuesUsingForEach(Map map) {
// Get all keys and values at the same time using map.forEach
print('----------');
print('Get keys and values using map.forEach:');
map.forEach((key, value) {
print('Key = $key : Value = $value');
});
}
// Output
Get keys and values using map.forEach:
Key = name : Value = Phuc Tran
Key = email : Value = phuc.tran@nextfunc.com
Key = website : Value = https://coflutter.com
4. Get all keys and values from Map using entries
void getKeysAndValuesUsingEntries(Map map) {
// Get all keys and values at the same time using entries
print('----------');
print('Get keys and values using entries:');
map.entries.forEach((entry) {
print('Key = ${entry.key} : Value = ${entry.value}');
});
}
// Output
Get keys and values using entries:
Key = name : Value = Phuc Tran
Key = email : Value = phuc.tran@nextfunc.com
Key = website : Value = https://coflutter.com
Full example (Github link)
void main() {
Map<String, String> map = {
'name': 'Phuc Tran',
'email': 'phuc.tran@nextfunc.com',
'website': 'https://coflutter.com'
};
getKeysFromMap(map);
getValuesFromMap(map);
getKeysAndValuesUsingForEach(map);
getKeysAndValuesUsingEntries(map);
}
void getKeysFromMap(Map map) {
print('----------');
print('Get keys:');
// Get all keys
map.keys.forEach((key) {
print(key);
});
}
void getValuesFromMap(Map map) {
// Get all values
print('----------');
print('Get values:');
map.values.forEach((value) {
print(value);
});
}
void getKeysAndValuesUsingForEach(Map map) {
// Get all keys and values at the same time using map.forEach
print('----------');
print('Get keys and values using map.forEach:');
map.forEach((key, value) {
print('Key = $key : Value = $value');
});
}
void getKeysAndValuesUsingEntries(Map map) {
// Get all keys and values at the same time using entries
print('----------');
print('Get keys and values using entries:');
map.entries.forEach((entry) {
print('Key = ${entry.key} : Value = ${entry.value}');
});
}
You may want to read
Dart – How to loop/iterate a list
Dart – How to find an item in a list
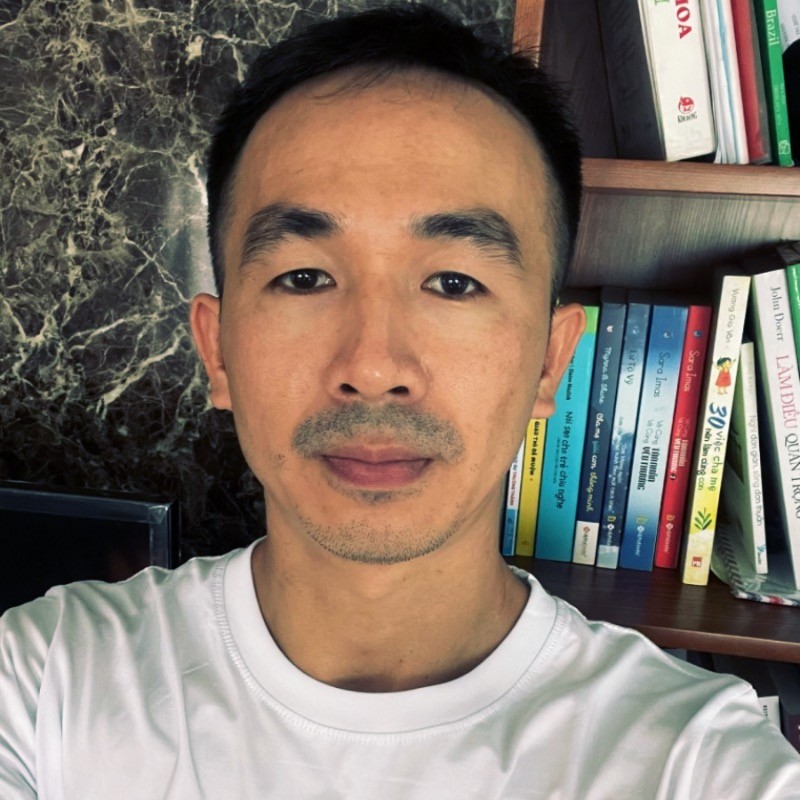
Creator of Coflutter.
Founder & CTO at Bumbii Technology
Founder at Bumbii K12
Follow him on Twitter, Github, StackOverflow, LinkedIn, Upwork.
Nice blog. Thank you so much.