Flutter support both material dialog (Android style) and Cupertino dialog (iOS).
To show a dialog, use showDialog( ) method:
void _showMaterialDialog() {
showDialog(
context: context,
builder: (context) {
return AlertDialog(
title: Text('Material Dialog'),
content: Text('Hey! I am Coflutter!'),
actions: <Widget>[
TextButton(
onPressed: () {
_dismissDialog();
},
child: Text('Close')),
TextButton(
onPressed: () {
print('HelloWorld!');
_dismissDialog();
},
child: Text('HelloWorld!'),
)
],
);
});
}
If you want to show iOS style dialog, Flutter provides that too:
void _showCupertinoDialog() {
showDialog(
context: context,
builder: (context) {
return CupertinoAlertDialog(
title: Text('Cupertino Dialog'),
content: Text('Hey! I am Coflutter!'),
actions: <Widget>[
TextButton(
onPressed: () {
_dismissDialog();
},
child: Text('Close')),
TextButton(
onPressed: () {
print('HelloWorld!');
_dismissDialog();
},
child: Text('HelloWorld!'),
)
],
);
});
}
Here is full example if you want to copy and run:
import 'package:flutter/material.dart';
import 'package:flutter/cupertino.dart';
void main() {
runApp(MyStatelessApp());
}
class MyStatelessApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Dialog Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: StatelessWidgetDemo(),
);
}
}
class StatelessWidgetDemo extends StatefulWidget {
@override
_StatelessWidgetDemoState createState() => _StatelessWidgetDemoState();
}
class _StatelessWidgetDemoState extends State<StatelessWidgetDemo> {
@override
Widget build(BuildContext context) {
return SafeArea(
child: Scaffold(
appBar: AppBar(
title: Text('Dialog Demo'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () {
_showMaterialDialog();
},
child: Text('Show Material Dialog'),
),
SizedBox(
height: 20,
),
ElevatedButton(
onPressed: () {
_showCupertinoDialog();
},
child: Text('Show Cupertino Dialog'),
),
SizedBox(
height: 20,
),
ElevatedButton(
onPressed: () {
_showSimpleDialog();
},
child: Text('Show Simple Dialog'),
)
],
),
)));
}
void _showMaterialDialog() {
showDialog(
context: context,
builder: (context) {
return AlertDialog(
title: Text('Material Dialog'),
content: Text('Hey! I am Coflutter!'),
actions: <Widget>[
TextButton(
onPressed: () {
_dismissDialog();
},
child: Text('Close')),
TextButton(
onPressed: () {
print('HelloWorld!');
_dismissDialog();
},
child: Text('HelloWorld!'),
)
],
);
});
}
_dismissDialog() {
Navigator.pop(context);
}
void _showCupertinoDialog() {
showDialog(
context: context,
builder: (context) {
return CupertinoAlertDialog(
title: Text('Cupertino Dialog'),
content: Text('Hey! I am Coflutter!'),
actions: <Widget>[
TextButton(
onPressed: () {
_dismissDialog();
},
child: Text('Close')),
TextButton(
onPressed: () {
print('HelloWorld!');
_dismissDialog();
},
child: Text('HelloWorld!'),
)
],
);
});
}
void _showSimpleDialog() {
showDialog(
context: context,
builder: (context) {
return SimpleDialog(
title: Text('Chosse an Option'),
children: <Widget>[
SimpleDialogOption(
onPressed: () {
_dismissDialog();
},
child: const Text('Option 1'),
),
SimpleDialogOption(
onPressed: () {
_dismissDialog();
},
child: const Text('Option 2'),
),
SimpleDialogOption(
onPressed: () {
_dismissDialog();
},
child: const Text('Option 3'),
),
SimpleDialogOption(
onPressed: () {
_dismissDialog();
},
child: const Text('Option 4'),
),
],
);
});
}
}
If you are looking for mobile/web Software Engineers to build you next projects, please Drop us your request!
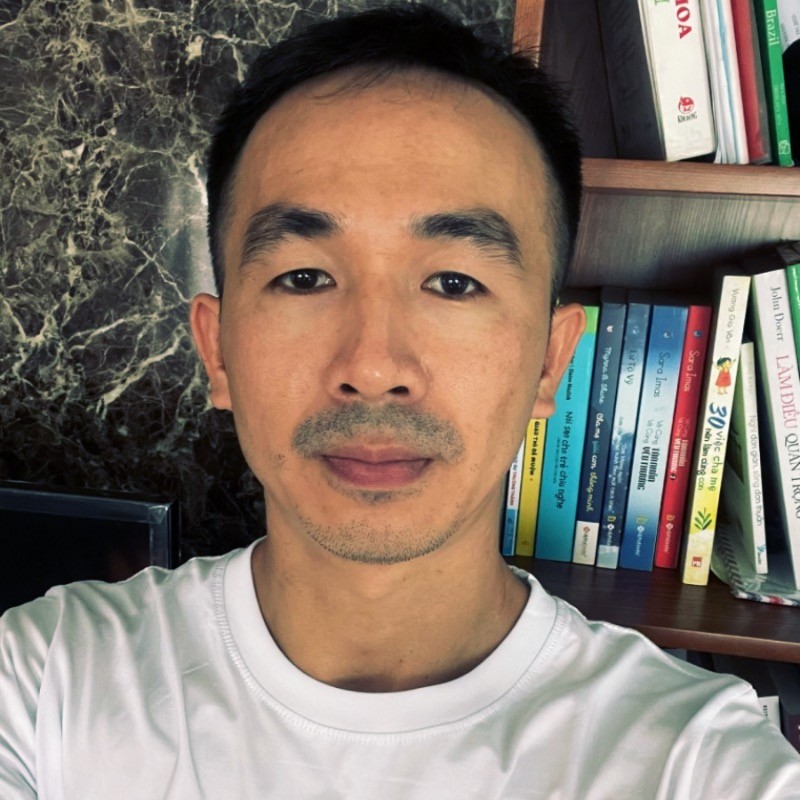
Creator of Coflutter.
Founder & CTO at Bumbii Technology
Founder at Bumbii K12
Follow him on Twitter, Github, StackOverflow, LinkedIn, Upwork.
import ‘package:flutter/cupertino.dart’;
import ‘package:flutter/material.dart’;
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: ‘Flutter Demo’,
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
final String title = “”;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
TextButton(
child: Text(‘Show Material Dialog’),
onPressed: _showMaterialDialog,
),
TextButton(
child: Text(‘Show Cupertino Dialog’),
onPressed: _showCupertinoDialog,
),
],
),
),
);
}
_showMaterialDialog() {
showDialog(
context: context,
builder: (_) => new AlertDialog(
title: new Text(“Material Dialog”),
content: new Text(“Hey! I’m Coflutter!”),
actions: <Widget>[
TextButton(
child: Text(‘Close me!’),
onPressed: () {
Navigator.of(context).pop();
},
)
],
));
}
_showCupertinoDialog() {
showDialog(
context: context,
builder: (_) => new CupertinoAlertDialog(
title: new Text(“Cupertino Dialog”),
content: new Text(“Hey! I’m Coflutter!”),
actions: <Widget>[
TextButton(
child: Text(‘Close me!’),
onPressed: () {
Navigator.of(context).pop();
},
)
],
));
}
}