To add padding to a String, Dart provides 2 methods: padLeft and padRight. This post shows you how those methods work through some simple examples.
Before show you the code, let’s analyze the specifications of padLeft (similar specifications are applied for padRight method):
Syntax:
String padLeft(int width, [String padding = ' ']);
1. This method return a string after applying below rules.
2. [width]: number of [padding] will be added on the left of original string.
– If [width] is smaller than or equal to the length of the original string, no padding is added.
– If a negative [width] is provided, it is treated as width = 0.
final coflutter = 'Coflutter'; // length = 9
print(coflutter.padLeft(3)); // width = 3 < 9 -> print: Coflutter
print(coflutter.padLeft(-10)); // width = - 10 < 0 -> print: Coflutter
3. The [padding] parameter is optional, if you skip it, the default padding is a one space character (‘ ‘). Please note that we are allowed to provide the [padding] which has length different from 1.
final coflutter = 'Coflutter'; // length = 9
// [width] = 12 -> padding length = 12 - 9 = 3
print(coflutter.padRight(12)); // print: Coflutter (3 spaces at the beginning)
print(coflutter.padRight(12, 'x')); // print: Coflutterxxx (3 'x' at the beginning)
print(coflutter.padRight(12, 'xx')); // print: Coflutterxxxxxx (3 'xx' at the beginning)
Put all together
main() {
final coflutter = 'Coflutter';
print(coflutter.padLeft(3));
print(coflutter.padLeft(-10));
print(coflutter.padLeft(12));
print(coflutter.padLeft(12, 'x'));
print(coflutter.padLeft(12, 'xx'));
}
Output
Coflutter
Coflutter
Coflutter
xxxCoflutter
xxxxxxCoflutter
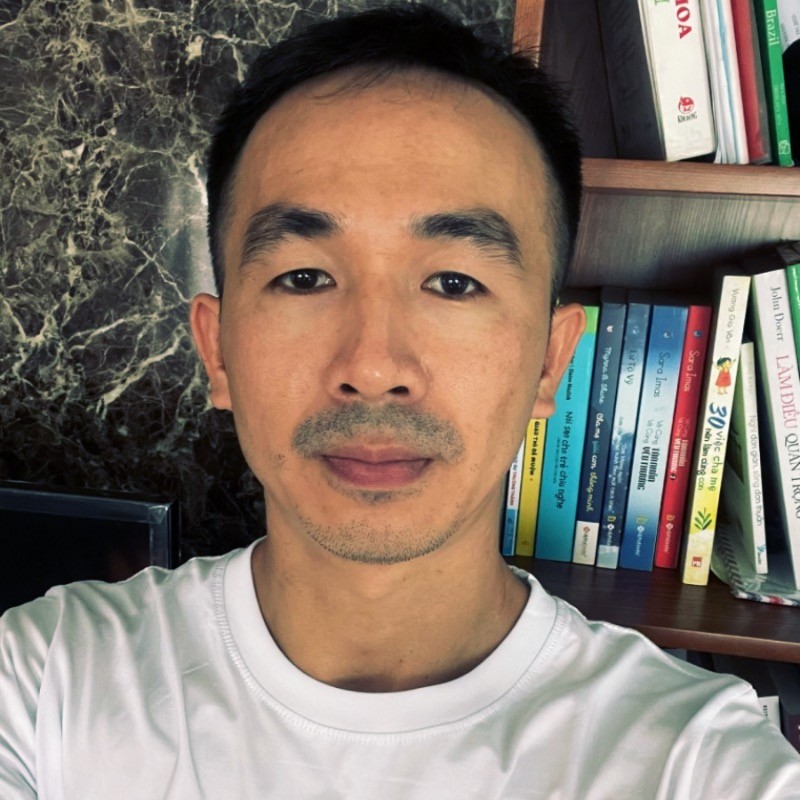
Creator of Coflutter.
Founder & CTO at Bumbii Technology
Founder at Bumbii K12
Follow him on Twitter, Github, StackOverflow, LinkedIn, Upwork.