Dart provides .values to get list of values in an enum. Since it is a list, we can use any method of List data type.
In this post we make a demo to loop through all the values of an enum.
enum Animal { Dog, Cat, Chicken, Elephant }
void main() {
Animal.values.forEach((name) {
print(name);
});
}
Output:
Animal.Dog
Animal.Cat
Animal.Chicken
Animal.Elephant
In case you want to print just the name (Dog, Cat, Chicken, Elephant), you can do one more step like below:
enum Animal { Dog, Cat, Chicken, Elephant }
void main() {
Animal.values.forEach((name) {
print(name?.toString()?.split('.')?.elementAt(1));
});
}
Output:
Dog
Cat
Chicken
Elephant
If you are looking for mobile/web Software Engineers to build you next projects, please Drop us your request!
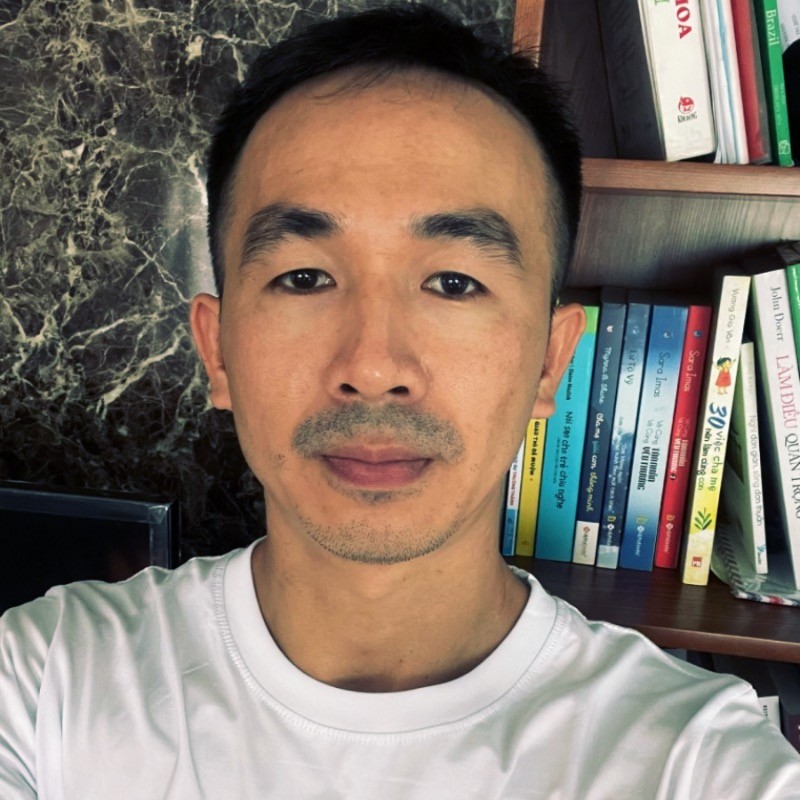
Creator of Coflutter.
Founder & CTO at Bumbii Technology
Founder at Bumbii K12
Follow him on Twitter, Github, StackOverflow, LinkedIn, Upwork.
how do you delete the “Animal.” ?