In this post, we will do a simple example to check if a String is a number.
1. Use tryParse method:
bool isNumericUsing_tryParse(String string) {
// Null or empty string is not a number
if (string == null || string.isEmpty) {
return false;
}
// Try to parse input string to number.
// Both integer and double work.
// Use int.tryParse if you want to check integer only.
// Use double.tryParse if you want to check double only.
final number = num.tryParse(string);
if (number == null) {
return false;
}
return true;
}
2. Use RegExp (Regular Expression)
bool isNumericUsingRegularExpression(String string) {
final numericRegex =
RegExp(r'^-?(([0-9]*)|(([0-9]*)\.([0-9]*)))$');
return numericRegex.hasMatch(string);
}
Test it!
void main() {
print(isNumericUsing_tryParse('12345')); // true
print(isNumericUsing_tryParse('12345a')); // false
print(isNumericUsing_tryParse('012345')); // true
print(isNumericUsing_tryParse('-12345')); // true
print(isNumericUsing_tryParse('1.23')); // true
print(isNumericUsing_tryParse('1.23f')); // false
print('---');
print(isNumericUsingRegularExpression('12345')); // true
print(isNumericUsingRegularExpression('12345a')); // false
print(isNumericUsingRegularExpression('012345')); // true
print(isNumericUsingRegularExpression('-12345')); // true
print(isNumericUsingRegularExpression('1.23')); // true
print(isNumericUsingRegularExpression('1.23f')); // false
}
If you are looking for mobile/web Software Engineers to build you next projects, please Drop us your request!
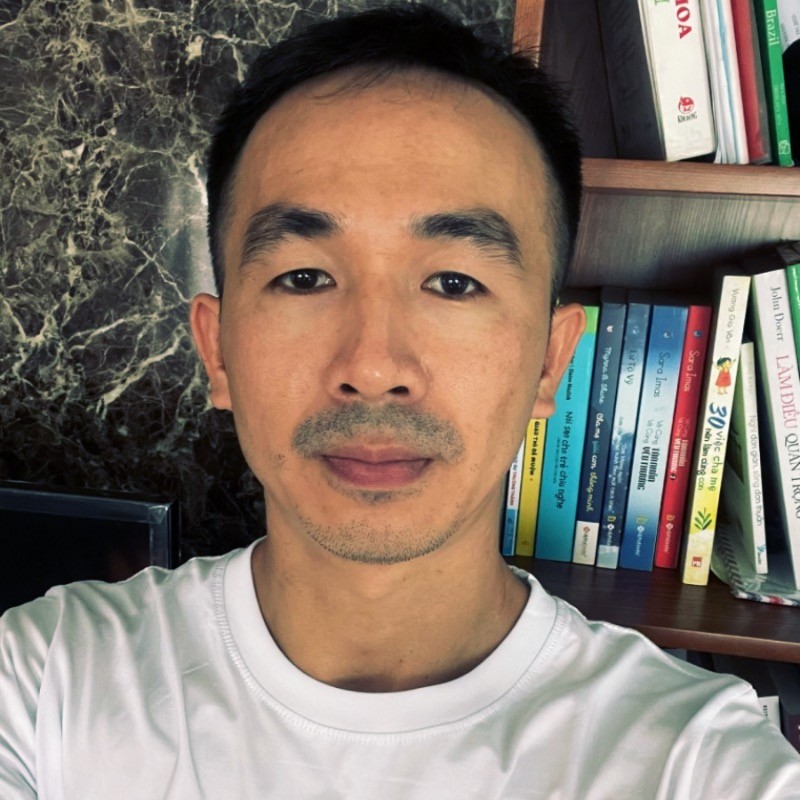
Creator of Coflutter.
Founder & CTO at Bumbii Technology
Founder at Bumbii K12
Follow him on Twitter, Github, StackOverflow, LinkedIn, Upwork.