In this post, we will create a Rounded Corners Image in Flutter by using ClipRRect.
import 'dart:io';
import 'package:flutter/material.dart';
class RoundedImage extends StatelessWidget {
final File imageFile;
final double? width;
final double? height;
final double radius;
const RoundedImage(
{Key? key,
required this.imageFile,
this.width = 100,
this.height = 100,
this.radius = 10})
: super(key: key);
@override
Widget build(BuildContext context) {
return ClipRRect(
borderRadius: BorderRadius.circular(radius),
child: Image.file(imageFile,
width: width, height: height,
// Crop center
fit: BoxFit.cover));
}
}
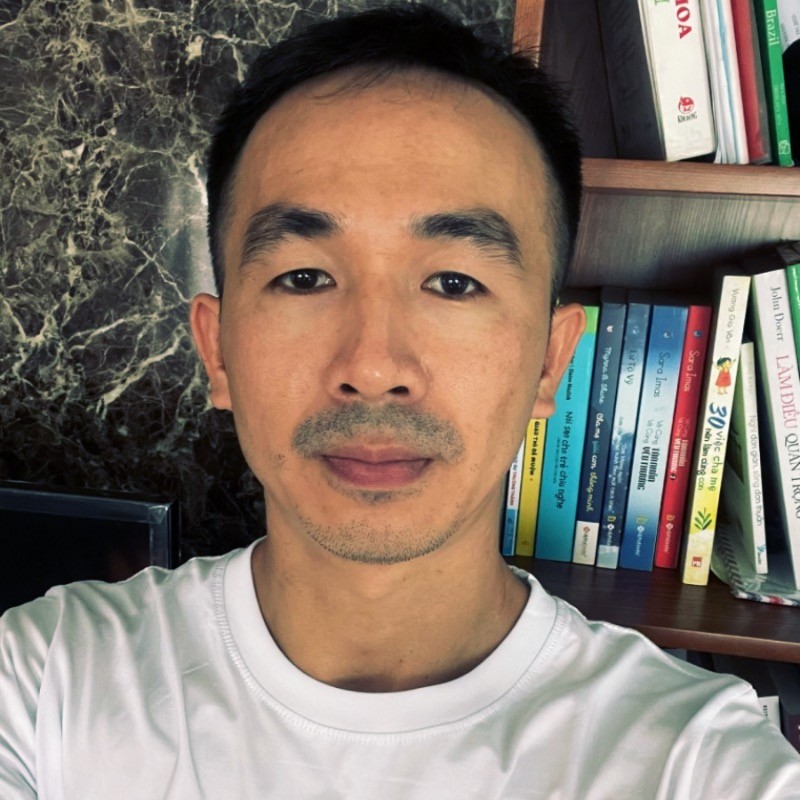
Creator of Coflutter.
Founder & CTO at Bumbii Technology
Founder at Bumbii K12
Follow him on Twitter, Github, StackOverflow, LinkedIn, Upwork.