As a developer, “calling” or “executing” functions is the thing you do many times per your working day. It’s a common action. However, in this post, we will try an uncommon way to execute functions in Dart using static Function.apply() method.
1. Syntax
dynamic apply (
Function function,
List? positionalArguments,
[Map<Symbol, dynamic>? namedArguments]
)
void helloCoflutter() {
print('Hello Coflutter.');
}
void main() {
Function.apply(helloCoflutter, [], {});
}
// Output
Hello Coflutter.
- function: The name of the function you want to execute (“helloCoflutter”).
- positionalArguments: List of (both required and optional) positional arguments , wrapped in square brackets. In above example, there is no positional argument, therefore we provide empty list ([ ]).
- namedArguments: Map of (optional) named arguments, wrapped in curly brackets. In above example, there is no named argument, therefore we provide empty map ({ }).
- The return type of Function.apply() is the return type of the executed function. In above example, the return type is void.
2. Examples
2a. Execute function with optional named parameters
void helloCoflutter1(int year, {String country, String city}) {
print('Hello Coflutter. $country, $city $year.');
}
void main() {
Function.apply(helloCoflutter1, [2020], {#country: 'Vietnam', #city: 'Ho Chi Minh'});
}
// Output
Hello Coflutter. Vietnam, Ho Chi Minh 2020.
- Function helloColutter1 has one required positional parameter (year) and two optional named parameters. The “year” is in square brackets, country and city are in curly brackets.
- Each optional named argument starts with a hash character (#)
2b. Execute function with optional positional parameters
void helloCoflutter2(int year, [String country, String city]) {
print('Hello Coflutter. $country, $city $year.');
}
void main() {
Function.apply(helloCoflutter2, [2020, 'Vietnam', 'Ho Chi Minh']);
}
// Output
Hello Coflutter. Vietnam, Ho Chi Minh 2020.
- All positional parameters (both required and optional) are in the square brackets.
2c. Execute function with return value
int sum(int a, int b) {
return a + b;
}
void main() {
int result = Function.apply(sum, [1, 2]);
print('Sum = $result');
}
// Output
Sum = 3
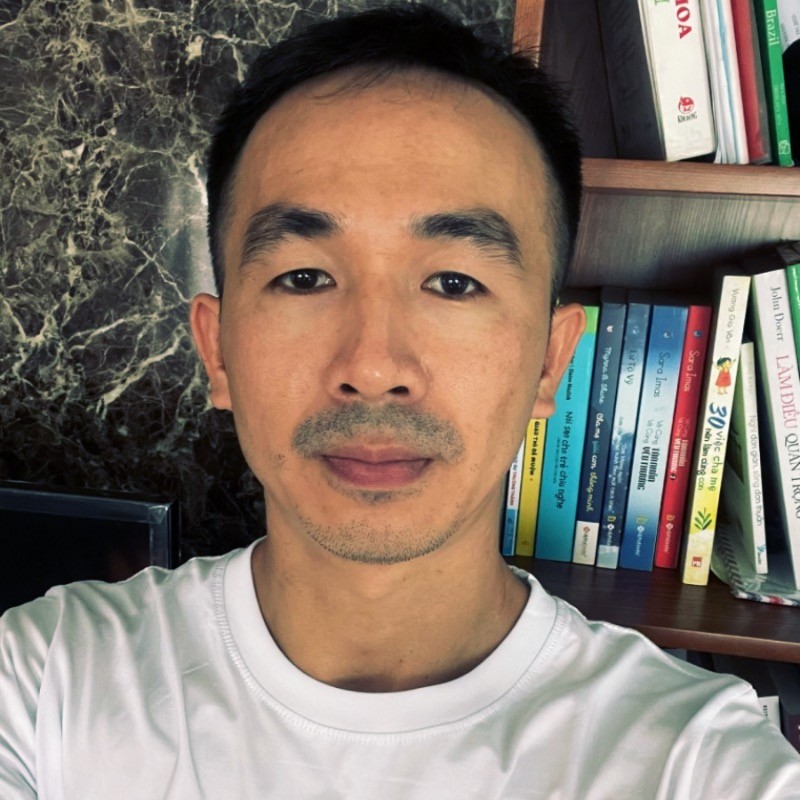
Creator of Coflutter.
Founder & CTO at Bumbii Technology
Founder at Bumbii K12
Follow him on Twitter, Github, StackOverflow, LinkedIn, Upwork.