Beside XML, JSON is a common text format that almost APIs use to return data to front end clients. When you develop Flutter or Dart apps, you may work with JSON frequently. In this post, we learn how to parse JSON in Dart/Flutter using Dart convert library (dart:convert).
Understand JSON structure
- JSON can contain object or array. An object in JSON is wrapped inside the braces { … }, while an array is wrapped inside square brackets [ … ].
{
"name": "John",
"age": 20
}
[
{
"name": "John",
"age": 20
},
{
"name": "Peter",
"age": 22
}
]
- JSON structures data by key-value. Key is always a string, but value could be anything (String, number, JSON object, JSON array…). This will affect the way we parse JSON in the next steps.
Parsing JSON
There are a few different approaches we can use to parse JSON: using dart:convert, using external packages on pub.dev (dart_json_mapper, json_annotation, json_serializable…). But in this post, I will show you how to parse JSON using dart:convert library only. Other approaches will be in other incoming articles.
1. Parse JSON Object
Suppose that we need to parse this JSON data:
{
"name": "John",
"age": 20
}
We create a Student class and do the parsing, pass the decoded JSON to the factory constructor:
class Student {
final String name;
final int age;
Student({this.name, this.age});
factory Student.fromJson(Map<String, dynamic> json) {
return Student(name: json['name'], age: json['age']);
}
// Override toString to have a beautiful log of student object
@override
String toString() {
return 'Student: {name = $name, age = $age}';
}
}
Use dart:convert to parse the JSON. Here I use “raw string” to represent the JSON text. If you don’t know about “raw string”, you can check point 4 and point 5 in String in Dart/Flutter – Things you should know.
void testParseJsonObject() {
final jsonString = r'''
{
"name": "John",
"age": 20
}
''';
// Use jsonDecode function to decode the JSON string
// I assume the JSON format is correct
final json = jsonDecode(jsonString);
final student = Student.fromJson(json);
print(student);
}
Test it
void main(List<String> args) {
testParseJsonObject();
}
// Output
Student: {name = John, age = 20}
2. Parse JSON Array
Similarly, we will parse JSON array here in this example. We reuse above Student class. Now we create a function to parse the array of students:
void testParseJSONArray() {
final jsonString = r'''
[
{
"name": "John",
"age": 20
},
{
"name": "Peter",
"age": 22
}
]
''';
// Use jsonDecode function to decode the JSON string
// I assume the JSON format is correct
final json = jsonDecode(jsonString);
// The JSON data is an array, so the decoded json is a list.
// We will do the loop through this list to parse objects.
if (json != null) {
json.forEach((element) {
final student = Student.fromJson(element);
print(student);
});
}
}
void main(List<String> args) {
testParseJSONArray();
}
// Output
Student: {name = John, age = 20}
Student: {name = Peter, age = 22}
If you are looking for a developer or a team to build you next projects, please Drop us your request!
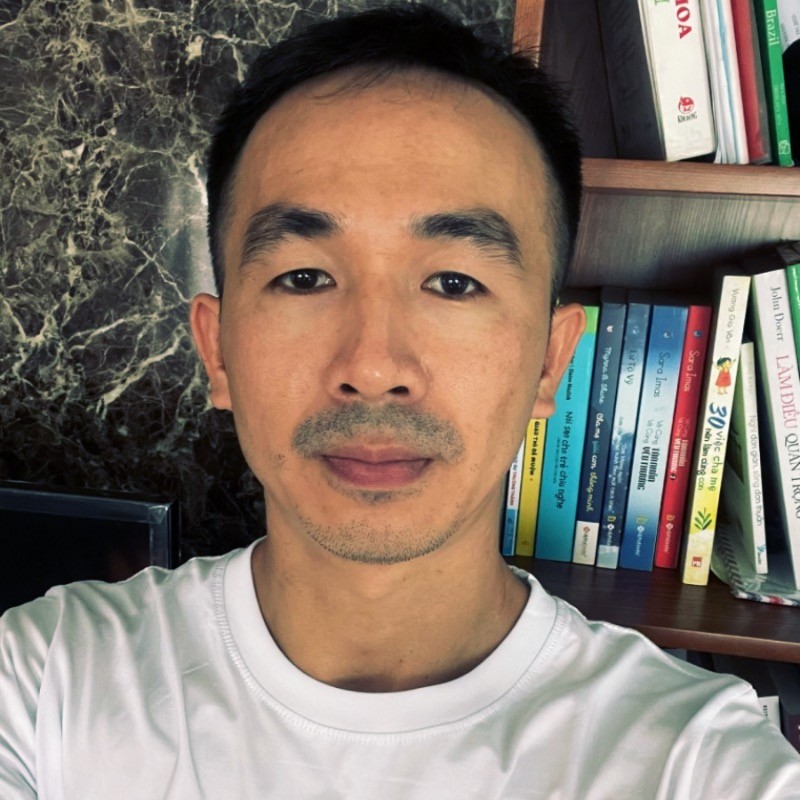
Creator of Coflutter.
Founder & CTO at Bumbii Technology
Founder at Bumbii K12
Follow him on Twitter, Github, StackOverflow, LinkedIn, Upwork.
My favorite tool is quicktype (https://app.quicktype.io/) to generate classes from JSON. Just paste the JSON and it will generate the class and the
toJson()
andfromJson()
functions, with two utility functions to use globally.When I have an API documentation with hundreds of JSON response strings, generating classes for them is extremely simplified by this tool.