In this post, we will create a demo to learn how to show and hide/dismiss soft keyboard in Flutter using FocusNode.
If you don’t want to go through step by step, you can use below method to save time:
/// Hide the soft keyboard.
void hideKeyboard(BuildContext context) {
FocusScope.of(context).requestFocus(FocusNode());
}
Here is what we are going to build:
I believe that the UI in this demo is quite simple, so I just focus on the methods to show and hide the keyboard. Final source code will be provided at the end of this post.
1. Declare and create new FocusNode
class _DismissKeyboardTutorialScreenState extends State<DismissKeyboardTutorialScreen> {
FocusNode focusNode;
@override
void initState() {
super.initState();
focusNode = FocusNode();
}
// ...
}
2. “Connect” your TextField with above focusNode
@override
Widget build(BuildContext context) {
// ... Other widgets
TextField(
focusNode: focusNode,
),
// ... Other widgets
}
3. Create showKeyboard() and dismissKeyboard() methods
void showKeyboard() {
focusNode.requestFocus();
}
void dismissKeyboard() {
focusNode.unfocus();
}
4. Use those methods
// ...
RaisedButton(
onPressed: () {
showKeyboard();
},
child: Text('Show Keyboard'),
),
RaisedButton(
onPressed: () {
dismissKeyboard();
},
child: Text('Dismiss Keyboard'),
),
// ...
5. Don’t forget to dispose the FocusNode
@override
void dispose() {
focusNode.dispose();
super.dispose();
}
– – – – –
Put it all together
import 'package:flutter/material.dart';
void main() => runApp(ShowSnackBarDemo());
class ShowSnackBarDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Coflutter - Dismiss Keyboard',
home: Scaffold(
appBar: AppBar(
title: const Text('Coflutter - Dismiss Keyboard'),
backgroundColor: const Color(0xffae00f0),
),
body: DismissKeyboardTutorialScreen(),
),
);
}
}
class DismissKeyboardTutorialScreen extends StatefulWidget {
@override
_DismissKeyboardTutorialScreenState createState() =>
_DismissKeyboardTutorialScreenState();
}
class _DismissKeyboardTutorialScreenState
extends State<DismissKeyboardTutorialScreen> {
FocusNode focusNode;
@override
void initState() {
super.initState();
focusNode = FocusNode();
focusNode.addListener(() {
print('Listener');
});
}
@override
void dispose() {
focusNode.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Padding(
padding: const EdgeInsets.all(10.0),
child: TextField(
autofocus: true,
focusNode: focusNode,
),
),
RaisedButton(
onPressed: () {
showKeyboard();
},
child: Text('Show Keyboard'),
),
RaisedButton(
onPressed: () {
dismissKeyboard();
},
child: Text('Dismiss Keyboard'),
),
],
),
);
}
void showKeyboard() {
focusNode.requestFocus();
}
void dismissKeyboard() {
focusNode.unfocus();
}
}
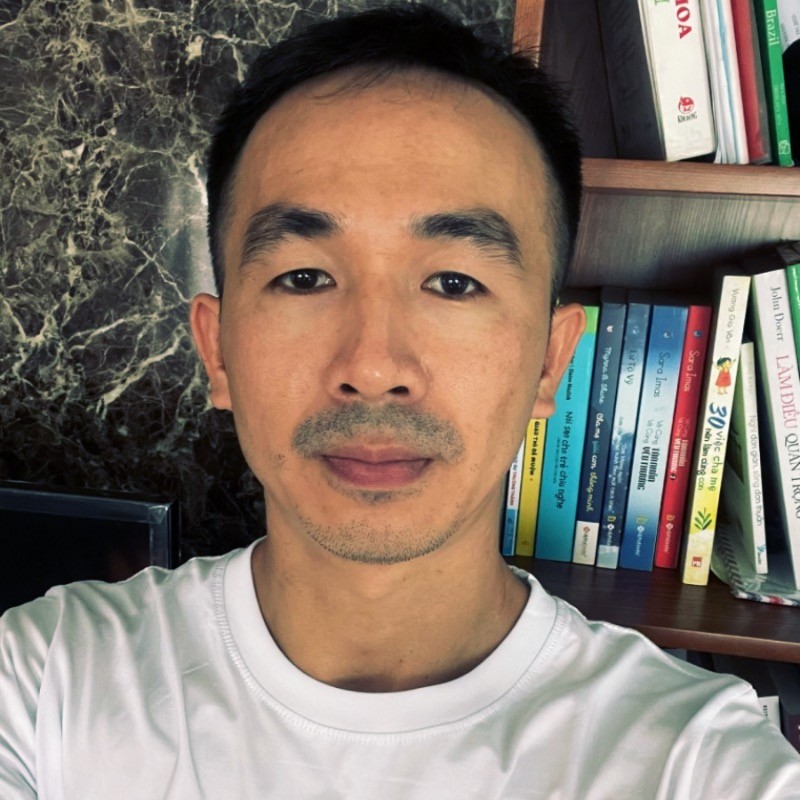
Creator of Coflutter.
Founder & CTO at Bumbii Technology
Founder at Bumbii K12
Follow him on Twitter, Github, StackOverflow, LinkedIn, Upwork.
Thanks for this post!