This post shows you an example of how to read the content of a file in Dart.
import 'dart:io';
main() {
final fileName = 'test.txt';
// Absolute path to the root folder of project
final rootPath = Directory.current.path;
// Absolute path to file
final path = rootPath+ '/lib/io/file/$fileName';
// Create a file, read the entire contents and print line by line
final file = File(path);
file.readAsString().then((value) => print(value));
// Read the file synchronously
final content = file.readAsStringSync();
print('readAsStringSync: $content');
// Print the stat of file
file.stat().then((value) => print(value));
}
Output
readAsStringSync: Coflutter - State management options:
Dart
Flutter
setState
MobX
Bloc
Redux
Provider
FileStat: type file
changed 2020-08-10 06:24:14.287
modified 2020-08-10 06:24:14.287
accessed 2020-08-10 06:24:14.907
mode rw-r--r--
size 85
Coflutter - State management options:
Dart
Flutter
setState
MobX
Bloc
Redux
Provider
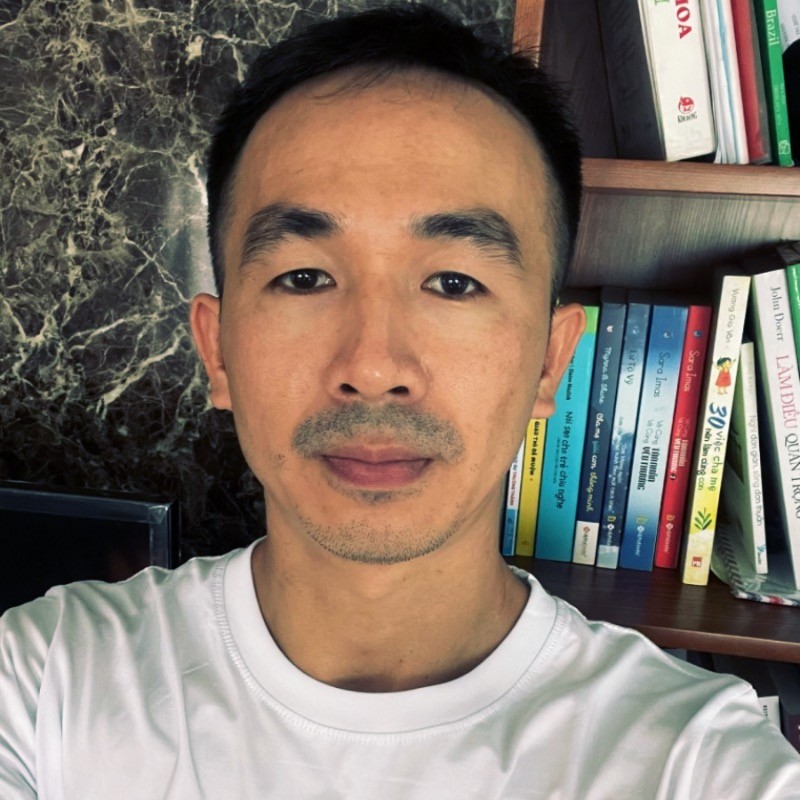
Creator of Coflutter.
Founder & CTO at Bumbii Technology
Founder at Bumbii K12
Follow him on Twitter, Github, StackOverflow, LinkedIn, Upwork.
good information