In this post, let’s learn how to convert or cast a double to an int and vice versa.
1. Convert double to int
To convert a double to int in Dart, we can use one of the built-in methods depending on your need:
toInt( ) or truncate( ): these 2 methods are equivalent, they both truncate the fractional/decimal part and return the integer part.
round( ): return the closest/nearest integer.
ceil( ): return the least integer that is not smaller than this number.
floor( ): return the greatest integer not greater than this number.
void main() {
double d = 10.3;
print(d.toInt()); // 10
print(d.truncate()); // 10
print(d.round()); // 10
print(d.ceil()); // 11
print(d.floor()); // 10
}
2. Convert int to double
So far, I know 2 ways to convert an integer to a double in Dart:
toDouble( ): as its name is saying, just convert the integer to a double
double.tryParse( ): provide an integer as a String to this method
void main() {
int i = 5;
print(i.toDouble()); // 5.0
print(double.tryParse('$i')); // 5.0
}
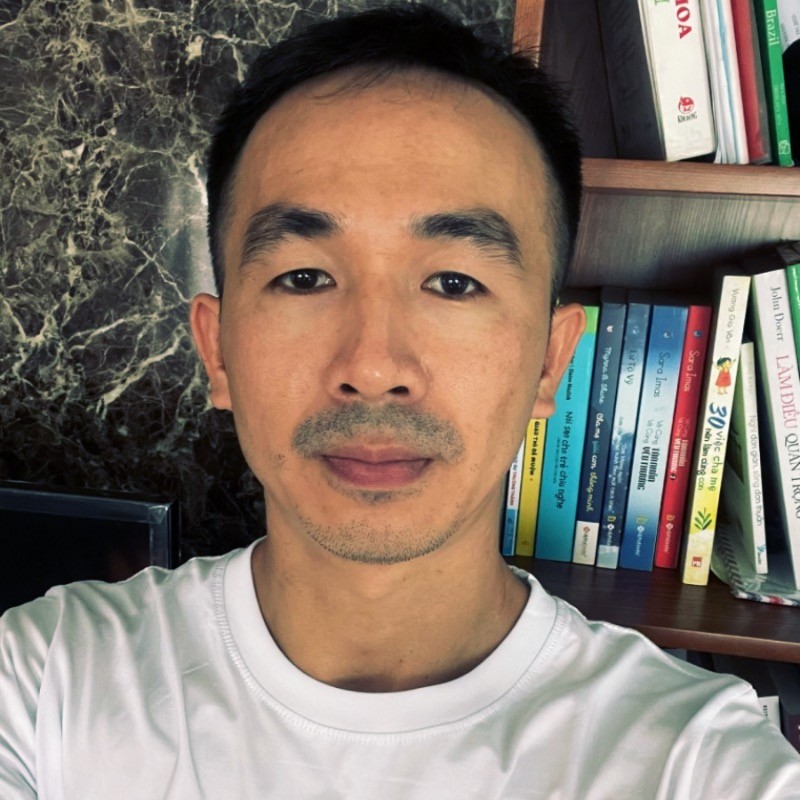
Creator of Coflutter.
Founder & CTO at Bumbii Technology
Founder at Bumbii K12
Follow him on Twitter, Github, StackOverflow, LinkedIn, Upwork.